- Mark as New
- Bookmark Message
- Subscribe to Message
- Mute Message
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Hi all,
I'm trying to expand my dashboard in a way that I don't need a submit Button anymore. Or let's better say, I still want it but in a different appearance.
Just look at this example code for a dashboard:
<form script="test_submit.js">
<label>test_submitform</label>
<fieldset submitButton="true">
<input type="text" token="field1">
<label>field1</label>
</input>
</fieldset>
<row>
<panel>
<html>
<a id="mysubmit">submit</a>
</html>
</panel>
</row>
<row>
<panel>
<table>
<search>
<query>| makeresults | eval field1="$field1$" | table field1</query>
<earliest>0</earliest>
<latest></latest>
</search>
</table>
</panel>
</row>
</form>
It is basically a dashboard (form) with one text input field that sets the token $field1$. The token is set after I click on the submit Button. Now I don't want to click the submit button but the Link in the HTML element instead.
I expanded my dashboard with some JavaScript code according to http://dev.splunk.com/view/SP-CAAAE4A . The code (test_submit.js) looks like this:
require([
"jquery",
"splunkjs/mvc/simpleform/formutils",
"splunkjs/mvc/simplexml/ready!"
], function ($,FormUtils) {
$("#mysubmit").on("click", function () {
FormUtils.submitForm();
});
});
This code worked in Splunk 6.4. Now in Splunk 6.5.0 it gives me the following error:
Chrome:
common.js:179 Uncaught TypeError: Cannot read property 'apply' of undefined
Firefox:
TypeError: (intermediate value).apply is undefined
Is this is bug in Splunk or in my code? And how can I work around this?
- Mark as New
- Bookmark Message
- Subscribe to Message
- Mute Message
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
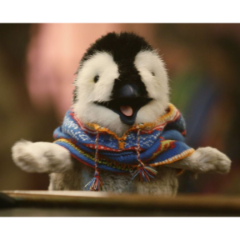
I would suggest going about this in JS without relying on the FormUtils class and edit the token values directly using the token models. The code would be as follows:
require([
'underscore',
'jquery',
"splunkjs/mvc",
"splunkjs/ready!",
"splunkjs/mvc/simplexml/ready!",
], function(_, $, mvc) {
function submitTokens() {
var submittedTokens = mvc.Components.get('submitted');
var defaultTokens = mvc.Components.get('default');
if (submittedTokens && defaultTokens) {
submittedTokens.set(defaultTokens.toJSON());
}
}
$("#mysubmit").on("click", function () {
submitTokens();
});
});
- Mark as New
- Bookmark Message
- Subscribe to Message
- Mute Message
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
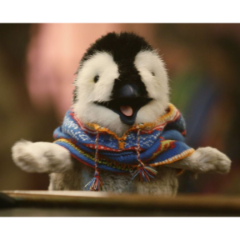
I would suggest going about this in JS without relying on the FormUtils class and edit the token values directly using the token models. The code would be as follows:
require([
'underscore',
'jquery',
"splunkjs/mvc",
"splunkjs/ready!",
"splunkjs/mvc/simplexml/ready!",
], function(_, $, mvc) {
function submitTokens() {
var submittedTokens = mvc.Components.get('submitted');
var defaultTokens = mvc.Components.get('default');
if (submittedTokens && defaultTokens) {
submittedTokens.set(defaultTokens.toJSON());
}
}
$("#mysubmit").on("click", function () {
submitTokens();
});
});
- Mark as New
- Bookmark Message
- Subscribe to Message
- Mute Message
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Thank you rjthibod. I guess that works for me.
- Mark as New
- Bookmark Message
- Subscribe to Message
- Mute Message
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
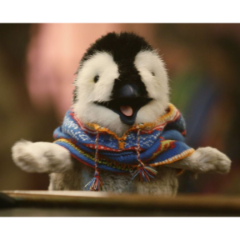
Sorry I couldn't give you a more direct answer to the exact cause of your problem. I made my recommendation because I know it works, and I always favor using the most direct mechanisms to deal with token and input classes given their consistency in 6.x. The wrapper-like classes like FormUtils seem to be more likely to change across versions.
